Something we can have the request to obscure sensible data for hiding purposes of information.
Pay attention that this is not a security prone method to hide sensible informations because someone can search on network requests and response and find what you’re trying to hide. We should do a true shadowing of the information on the BE, that delivers that information.
The inability to see the data on the screen is useful if you are in a public place. Someone passing by might see otherwise confidential information that we already know and don’t need to know.
The function we will see creates an array of the length of the characters covered and aggregates them into a single string of asterisks.
/**
* @description Return an obscured string.
* @param visibleData "Visible string to obscure."
* @param show [optional] "number of visible characters on total length."
*/
function obscureData(visibleData, show = 3) {
return (visibleData && visibleData.length > show) ?
`*${new Array(visibleData.length - show).join('*')}${visibleData.substr(-show)}`
: '********';
}
In addition, we also an optional parameter to decide how many characters we should make visible (the default is 3). This partial blackout can be useful for phone numbers for which you don’t want to show all the data, unless requested.
A generic string “********
” is returned if the string, passed in input, is shorter than the number of characters to show.
Obscure sensible data or show them
Now let’s say we want to allow to show the data temporarily hidden on the screen. For our example we have two phone numbers with two buttons next to each.
<div class="text-center mb-3">
<span id="hide-data-1" class="hide-data">3331111111</span>
<button class="btn-primary ms-3" onclick="toggleHideData('hide-data-1')">Show/hide</button>
</div>
<div class="text-center">
<span id="hide-data-2" class="hide-data">333222222</span>
<button class="btn-primary ms-3" onclick="toggleHideData('hide-data-2')">Show/hide</button>
</div>
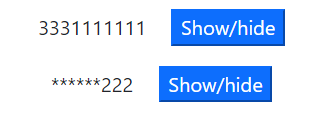
To act on multiple fields, we apply a hide-data
class to each element whose text is to be hidden and loop through them. For each occurrence of the loop we use the blanking function, seen at the beginning.
document.querySelectorAll('.hide-data').forEach(i => {
// Add data-hide attribute with text content to every element to hide.
i.dataset.hide = i.textContent;
// Hide its text content.
i.textContent = obscureData(i.textContent);
});
Once this is done, we apply the onclick=""
trigger to the buttons with the function to show or hide the data. As a parameter in the function we use the element to hide or show the text.
<button class="btn-primary ms-3" onclick="toggleHideData('id-of-text-element-to-hide')">Show/hide</button>
Once this is done, all we need is the function of the button.
This function is based on the presence or absence of the data attribute in the element whose id we passed. If it finds it, it passes its value to the content text. Otherwise (in the else) apply that text to the data-attribute and then obscure the text with the already seen function.
function toggleHideData(id) {
const hideData = document.getElementById(id);
// Show text content.
if (hideData.dataset.hide) {
hideData.textContent = hideData.dataset.hide;
delete hideData.dataset.hide;
// Hide text content.
} else {
hideData.dataset.hide = hideData.textContent;
hideData.textContent = obscureData(hideData.textContent);
}
}
If you’re working in angular and need a way to hide or show the password when needed, I recommend this targeted article instead:
Easy way to show/hide a password field in Angular
Here is a working example of the basic dimming case.
That’s all to obscure sensible data.
Try it at home!