Let’s create REST services with Drupal.
You may need to export the power of Drupal to deliver content to multiple systems. This possibly even in multiple different technologies. In these cases it can be useful to use a more generic language to transmit this content, such as JSON.
Drupal allows the delivery of content not only towards its Live part but also in “Headless” mode, in the format you prefer.
If you want to provide simple lists of contents, it is often convenient to rely on the “Views” module which serves precisely for this purpose. They provide a graphical interface that allows customizations that are also quite important on how you want to provide the information and context queries you want to define.
If, on the other hand, you want even greater flexibility on what you display, you can use the REST resources. They can be provided with Drupal.
What modules to install
To do this you need, obviously apart from the completed installation of Drupal, 3 basic modules installed:
- REST UI: provides a user interface to manage REST resources.
- RESTful Web Services: exposes entities and other resources as RESTful web API.
- Serialization: provides a service for (de)serializing data to/from formats such as JSON and XML.
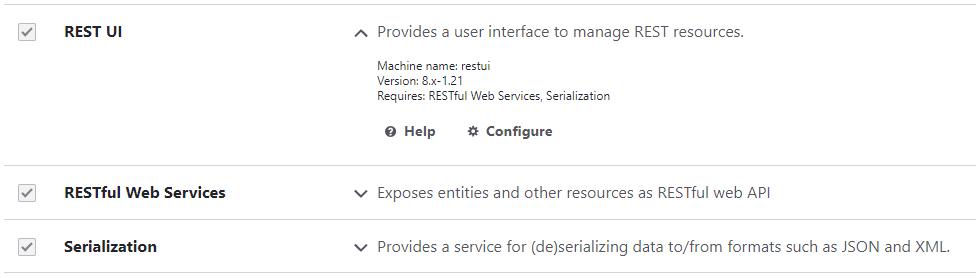
Once you have these 3 modules installed, you will need to create your own module. It is for the insertion of the resources, which we will do later.
To do this we create two files, inside the “modules” folder and preferably in a “custom” sub-folder:
- custom_module_name.info.yml
- custom_module_name.module
In the first we insert the information of our form.
# custom_module_name.info.yml
name: CUSTOM MODULE
type: module
description: "To manage REST resources."
package: CUSTOM MODULE PACKAGE
core_version_requirement: ^8 || ^9 || ^10
dependencies:
- drupal:rest
# Information added by Drupal.org packaging script on 2022-07-29
version: '9.x-1.0'
project: 'custom_module_name'
datestamp: 1659086916
In the second we insert what will be needed by our module, for our purposes nothing is needed inside this file.
<?php
// custom_module_name.module
/**
* @file
* Code for the Idro custom module.
*/
Script part for REST services
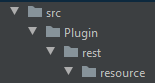
Our resource plugin will be installed inside our custom module, under the tree:src -> Plugin -> rest -> resource
This plugin will be a php file containing the resource class, with the @RestResource
annotation with some necessary information, including the path (in our case “/api/my-rest-api“).
<?php
// MyCustomResource.php
namespace Drupal\custom_module_name\Plugin\rest\resource;
use Drupal\rest\Plugin\ResourceBase;
use Drupal\rest\ResourceResponse;
/**
* Provides an Api Resource.
*
* @RestResource(
* id = "api_resource",
* label = @Translation("Api Resource"),
* uri_paths = {
* "canonical" = "/api/my-rest-api"
* }
* )
*/
class MyCustomResource extends ResourceBase {
/**
* Responds to entity GET requests.
* @return \Drupal\rest\ResourceResponse
*/
public function get() {
$all_result = $node_result = null;
///////////////////////////////////////////////
// QUERY PART.
$nodeQuery = \Drupal::entityQuery('node');
$nids = $nodeQuery->condition('type', 'my_custom_content_type')->execute();
if (is_array($nids)) {
$nodes = \Drupal\node\Entity\Node::loadMultiple($nids);
///////////////////////////////////////////////
// LOOP PART.
foreach ($nodes as $node) {
$node_result[] = array(
'id' => (int) $node->nid->value,
'name' => $node->field_name->value,
'title' => $node->title->value,
);
}
///////////////////////////////////////////////
// WRAP PART.
$all_result = array(
'result' => array(
'items' => $node_result,
),
);
}
$response = new ResourceResponse($all_result);
$response->addCacheableDependency($all_result);
return $response;
}
}
Activate your new services
Once you will have created the resource, you will have to go and activate it at the path /admin/config/services/rest , looking for your new service. Try to clean the cache, at the path /admin/config/development/performance , in case you don’t see your newly created service.
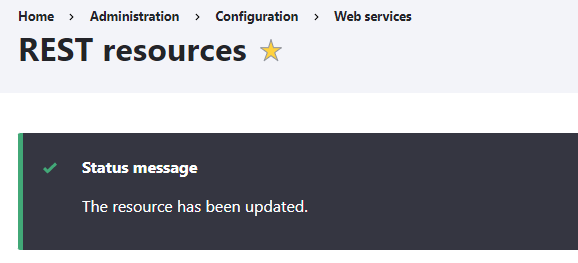
That’s all, with the REST services in Drupal.
Try it at home!