Often in level games, in very long levels, it can be useful that the user does not go back to the beginning of the game every time and has to re-face any monster already faced but respawns at the last registered checkpoint, with some of the parts already brought to term. With this tutorial you can make a checkpoint system for levels in Unity games.
For very simple games or levels it would be enough to restart the scene using the following command.
SceneManager.LoadScene(SceneManager.GetActiveScene().name);
With more complex games, the game becomes more complicated and with it the duration of the levels.
To do this, some checkpoints can be placed here and there to ensure that if our user reaches one, his position and his “status” at that precise moment are recorded and he does not have to start over.
The Prefab
of our player must have a specific tag in order to be recognized by the checkpoint.
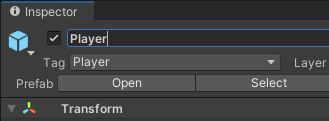
The checkpoint must have a Collider
component associated with the Is Trigger
flag enabled (in order not to block the passage to our player). In addition to this, it must have associated the script for saving the last recorded position.
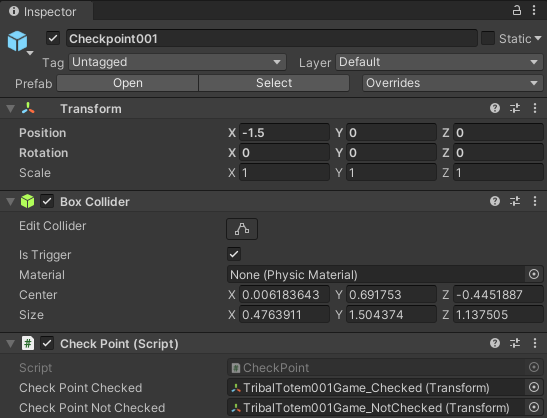
Optionally we can insert a cosmetic change to our checkpoint once the player has passed through it.
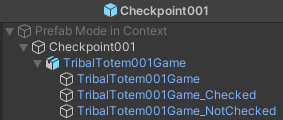
These variants of the checkpoint will be inserted into the script.

In my case I wanted to insert colored rags once the player passes the tribal totem.
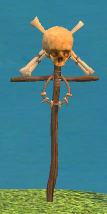
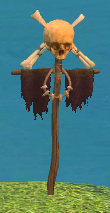
Let’s make code for the checkpoint system!
Now we come to the heart of our system, the script that records the player’s position. This position may be based on the player’s position at the time of contact with the trigger.
// CheckPoint.cs
using UnityEngine;
public class CheckPoint : MonoBehaviour {
[SerializeField] private Transform checkPointChecked;
[SerializeField] private Transform checkPointNotChecked;
private void Start() {
checkPointChecked.GetComponent<Renderer>().enabled = false;
checkPointNotChecked.GetComponent<Renderer>().enabled = false;
}
private void OnTriggerEnter(Collider other) {
if (other.gameObject.CompareTag("Player")) {
GameManager.lastCheckPointPosition =
new Vector3(other.gameObject.transform.position.x, other.gameObject.transform.position.y, other.gameObject.transform.position.z);
// GameManager.lastCheckPointPosition = new Vector3(transform.position.x, transform.position.y, transform.position.z);
GameManager.doblonsCheckpointCount = GameManager.doblonsCount;
// checkPointChecked.GetComponent<Renderer>().enabled = true;
checkPointNotChecked.GetComponent<Renderer>().enabled = true;
}
}
}
The manager of our game will have to save the information of the last available position of the player upon contact with the checkpoint. This position will be updated every time the player approaches another checkpoint.
// GameManager.cs
using UnityEngine;
public class GameManager : MonoBehaviour {
public static Vector3 lastCheckPointPosition = Vector3.zero;
public static void resetLevel()
{
lastCheckPointPosition = Vector3.zero;
}
}
Clearly, when exiting the level for any reason, our player will have to go back to the starting point.
At this point it will be necessary to act on the script of our Player
, specifically in its method of restarting the game.
// Player.cs
using UnityEngine;
// using UnityEngine.SceneManagement;
public class Player : MonoBehaviour {
Respawn() {
// Check for Player position different from start.
if (GameManager.lastCheckPointPosition != Vector3.zero) {
startPosition = transform.position = GameManager.lastCheckPointPosition;
} else {
transform.position = startPosition;
}
// SceneManager.LoadScene(SceneManager.GetActiveScene().name);
}
}
You can replace re-loading the scene with restarting from the last known position.
Now you can create your own checkpoint system and have your characters start over wherever you like, in your Unity games.
Try it at home!