A library is a reusable plugin, to help us in some tasks within our project.
We can start by simply using this command:
ng generate library calc-lib
And generate our library in project, named calc-lib
in this case.
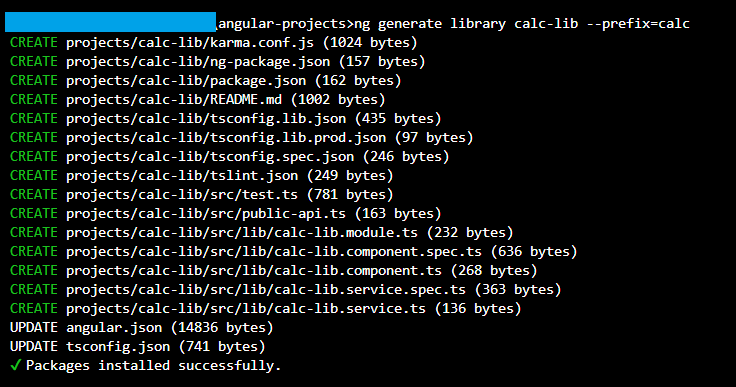
With it, we’ll see our angular.json modiefied with one more project of type: library.
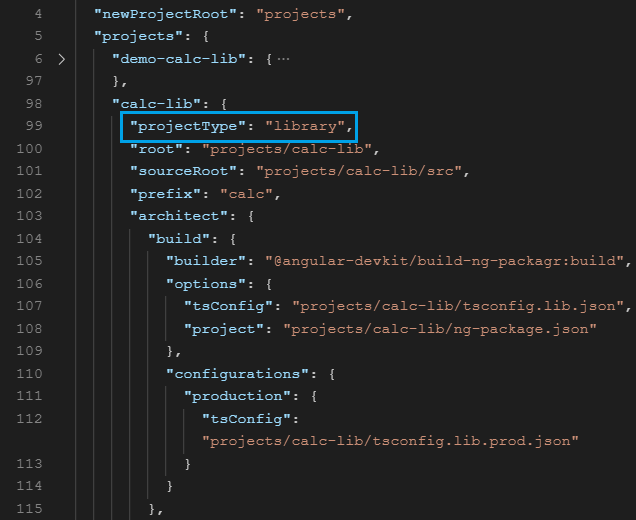
It will also add the library interface to export our library component, the public-api.ts
export * from './lib/calc-lib.service';
export * from './lib/calc-lib.component';
export * from './lib/calc-lib.module';
That command will also add the ng-packagr
in our package.json
to handle our library.
Now we start to create our example library component:
// calc-lib.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'calc-lib',
template: `
<div>
<label for="v1">Val 1:</label><input #v1 id="v1" type="number" />
<label for="v2">Val 2:</label><input #v2 id="v2" type="number" />
<p>
{{ result }}
</p>
<button (click)="add(v1.value, v2.value)">Add</button>
<button (click)="subtract(v1.value, v2.value)">Subtract</button>
<button (click)="multiply(v1.value, v2.value)">Multiply</button>
<button (click)="divide(v1.value, v2.value)">Divide</button>
</div>
`,
styles: []
})
export class CalcLibComponent implements OnInit {
result: number;
constructor() { }
ngOnInit(): void {
}
add(val1, val2) {
this.result = parseFloat(val1) + parseFloat(val2);
}
subtract(val1, val2) {
this.result = parseFloat(val1)- parseFloat(val2);
}
multiply(val1: number, val2: number) {
this.result = val1 * val2;
}
divide(val1: number, val2: number) {
if (val2 == 0) this.result = undefined;
this.result = val1 / val2;
}
}
You can import directly your library project in your using project module, after you have built it.
// app.module.ts
import { CalcLibModule } from 'calc-lib';
...
imports: [
CalcLibModule,
BrowserModule,
AppRoutingModule
],
...
This because the angular scaffolding command added the path pointing in your main tsconfig.json:
// tsconfig.json
..
"calc-lib": [
"dist/calc-lib/calc-lib",
"dist/calc-lib"
]
..
And we can use our new created library component in other using components.
// app.component.html
<calc-lib></calc-lib>
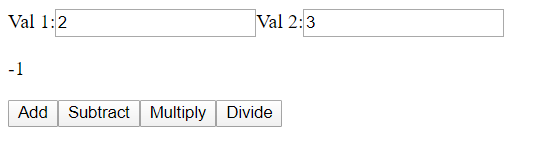
Try it at home!